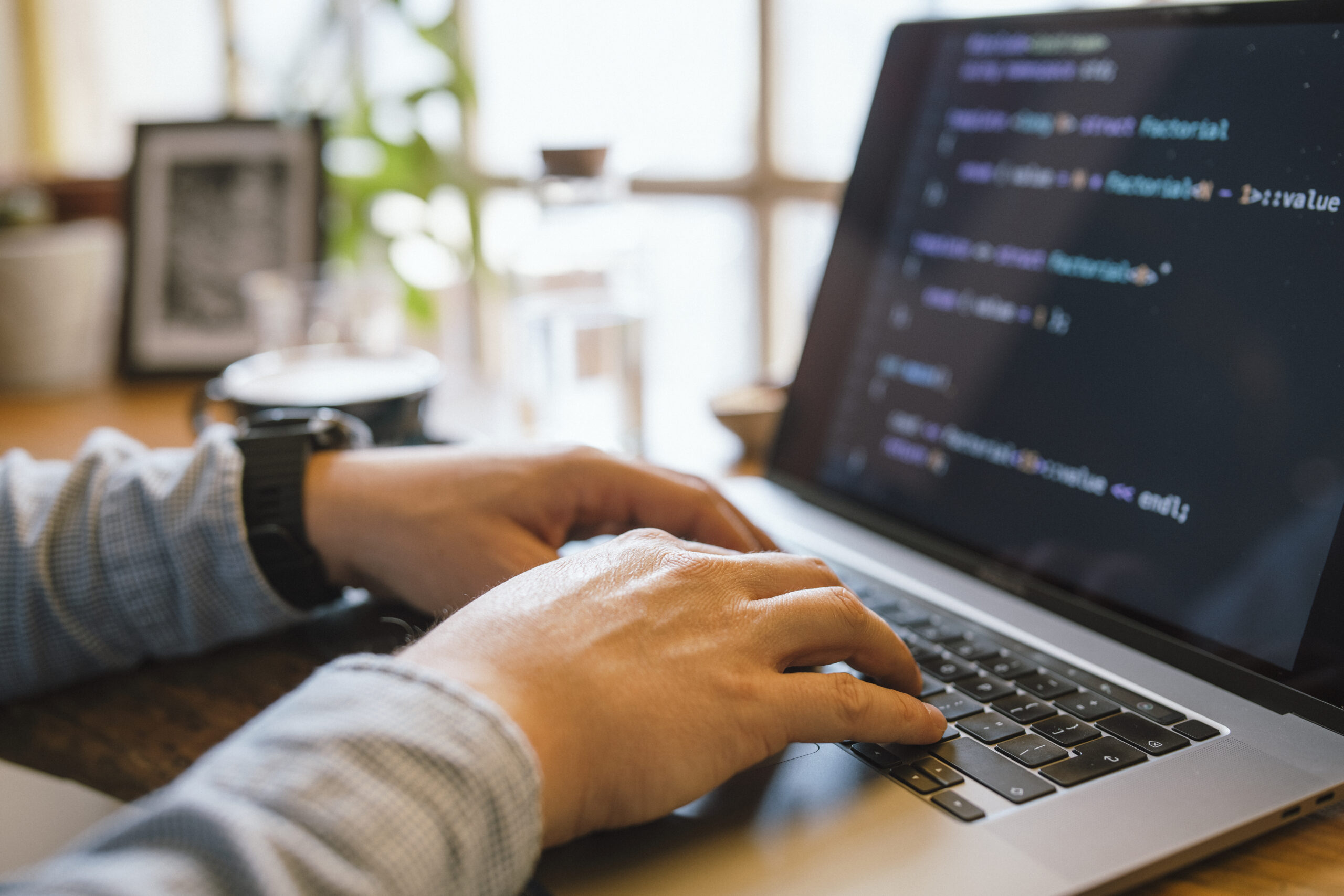
Debugging is Among the most critical — however usually overlooked — expertise within a developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Feel methodically to resolve difficulties proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous approaches to help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Although creating code is 1 part of development, recognizing tips on how to communicate with it effectively all through execution is Similarly crucial. Contemporary enhancement environments appear Outfitted with potent debugging abilities — but several developers only scratch the floor of what these resources can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code on the fly. When applied properly, they Permit you to observe particularly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, check community requests, see true-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI troubles into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above functioning processes and memory management. Mastering these tools could have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate units like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement setting making sure that when challenges crop up, you’re not shed at the hours of darkness. The better you realize your tools, the more time you are able to invest solving the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most vital — and often ignored — steps in effective debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to create a dependable ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as you can. Inquire questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug happens.
Once you’ve gathered enough facts, make an effort to recreate the problem in your neighborhood environment. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, take into consideration producing automatic exams that replicate the sting scenarios or state transitions included. These tests not simply assist expose the situation but additionally protect against regressions in the future.
From time to time, the issue could be natural environment-particular — it would transpire only on certain working devices, browsers, or under certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t simply a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But after you can continually recreate the bug, you might be now midway to fixing it. By using a reproducible circumstance, You may use your debugging tools more successfully, check prospective fixes securely, and talk a lot more Obviously using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s where by builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are sometimes the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as aggravating interruptions, developers should master to take care of error messages as direct communications from the procedure. They usually tell you exactly what transpired, wherever it occurred, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the information thoroughly and in full. Quite a few developers, especially when underneath time strain, glance at the main line and promptly begin making assumptions. But further within the mistake stack or logs could lie the true root bring about. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a particular file and line range? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to acknowledge these can drastically quicken your debugging course of action.
Some errors are obscure or generic, As well as in those circumstances, it’s important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede more substantial difficulties and supply hints about possible bugs.
Eventually, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info throughout improvement, INFO for typical situations (like prosperous start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise complications, and Deadly once the system can’t go on.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your method. Deal with critical activities, state improvements, input/output values, and important final decision points in the code.
Format your log messages clearly and continually. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a effectively-thought-out logging technique, you could reduce the time it will require to identify problems, achieve further visibility into your applications, and Enhance the All round maintainability and trustworthiness of your code.
Consider Similar to a Detective
Debugging is not just a specialized undertaking—it is a type of investigation. To properly detect and repair bugs, developers have to solution the process like a detective fixing a thriller. This mentality assists stop working elaborate problems into manageable elements and observe clues logically to uncover the root trigger.
Start off by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Similar to a detective surveys a criminal offense scene, accumulate just as much applicable information and facts as you could without leaping to conclusions. Use logs, exam cases, and person experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Request your self: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this situation occurred before less than identical situation? The aim is always to narrow down options and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled ecosystem. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often cover within the the very least anticipated sites—like a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Short term fixes may conceal the actual issue, just for it to resurface later.
And finally, continue to keep notes on Everything you tried out and discovered. check here Just as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering hidden problems in intricate units.
Write Exams
Composing assessments is among the simplest methods to boost your debugging capabilities and In general development efficiency. Exams not merely support capture bugs early and also function a security Web that offers you assurance when making modifications for your codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly where by and when a dilemma takes place.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically lessening enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—troubles that reappear soon after Formerly being preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist make sure several areas of your application get the job done collectively smoothly. They’re significantly valuable for catching bugs that take place in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you would like to comprehend its inputs, envisioned outputs, and edge circumstances. This level of comprehension naturally sales opportunities to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game into a structured and predictable method—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough issue, it’s straightforward to become immersed in the situation—gazing your monitor for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is simply stepping absent. Taking breaks helps you reset your mind, decrease disappointment, and often see the issue from a new perspective.
When you're too close to the code for too lengthy, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to a different activity for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma when they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also aid prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You may instantly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nonetheless it basically contributes to a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak spot—it’s a smart method. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel vision that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful in case you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in the workflow or understanding and assist you to Develop stronger coding habits shifting forward.
Documenting bugs can also be an excellent habit. Keep a developer journal or preserve a log where you note down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see styles—recurring difficulties or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Understanding culture.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.